JavaScript, often abbreviated as JS, is a high-level, interpreted scripting language that enables developers to add functionality to web pages. It’s a client-side scripting language, meaning it runs in a user’s web browser and interacts with the Document Object Model (DOM) to manipulate web content dynamically.
Introduction to JS
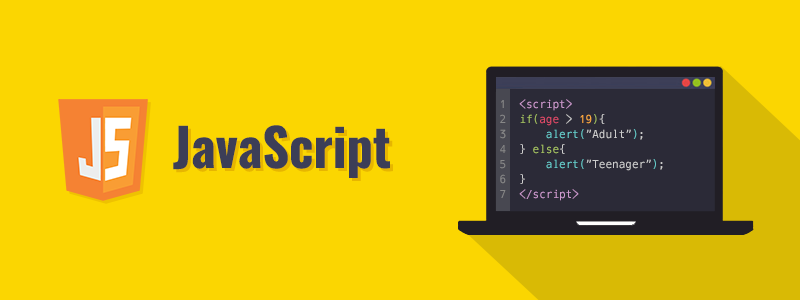
1. Birth of JavaScript (1995):
- JavaScript was created by Brendan Eich, a programmer at Netscape Communications Corporation, in 1995. It was originally called “Mocha” and later “LiveScript” before being officially named JavaScript.
- The initial goal was to develop a scripting language for Netscape’s web browser, Navigator, to add interactivity to static web pages.
2. Purpose of JavaScript:
- JavaScript is used to enhance the interactivity and user experience of websites. It allows developers to create features like interactive forms, animations, real-time updates, and more.
3. Features of JavaScript:
- Versatile: JavaScript can be used for various purposes, including web development, server-side scripting (Node.js), and even mobile app development (React Native).
- Event-Driven: JavaScript responds to user actions or events, making it suitable for creating interactive web pages.
- Lightweight: JavaScript files are typically small, so they load quickly in web browsers.
JavaScript Basics
Concept | Description | Example |
---|---|---|
Variables | Containers for storing data. | var name = “John”; |
Data Types | Categories of data values in JavaScript. | – Primitive Types: Number, String, Boolean, null, undefined, Symbol. – Reference Types: Object (including Arrays and Functions). |
Operators | Symbols used for operations on data. | var sum = x + y; var isGreater = x > y; |
Conditional Statements | Used for decision-making based on conditions. | javascript if (age >= 18) { console.log(“Adult”); } else { console.log(“Minor”); } |
Loops | Control structures for iterating through data. | javascript for (var i = 0; i < 5; i++) { console.log(“Iteration ” + i); } |
Functions | Reusable blocks of code. | javascript function greet(name) { console.log(“Hello, ” + name); } |
Working with the Document Object Model (DOM)
- Accessing DOM Elements:
- You can access HTML elements in the DOM using various methods, such as
getElementById
,querySelector
,querySelectorAll
, and more. For example:
var elementById = document.getElementById(“myId”);
var elementBySelector = document.querySelector(“.myClass”); - You can access HTML elements in the DOM using various methods, such as
- Modifying Element Properties:
- You can change the content, attributes, and styles of DOM elements using JavaScript. For example:
elementById.innerHTML = “New content”;
elementById.setAttribute(“class”, “newClass”);
elementById.style.color = “blue”; - Event Handling:
- JavaScript allows you to attach event listeners to DOM elements to respond to user interactions, such as clicks, mouse movements, and keyboard input. For example:
elementById.addEventListener(“click”, function() {
alert(“Element clicked!”);
}); - Creating and Appending Elements:
- You can create new HTML elements dynamically and add them to the DOM using methods like
createElement
andappendChild
. For example:
var newDiv = document.createElement(“div”);
newDiv.innerHTML = “New element”;
document.body.appendChild(newDiv); - You can create new HTML elements dynamically and add them to the DOM using methods like
JavaScript Objects and Data Structures
Object or Data Structure | Description | Example |
---|---|---|
Variables | Containers for storing data. | javascript var age = 30; let name = "John"; const PI = 3.14; |
Data Types | Categories of data values in JavaScript. | – Primitive Types: Number, String, Boolean, null, undefined, Symbol. – Reference Types: Object (including Arrays, Functions, and Custom Objects). |
Arrays | Ordered collections of values, often used for lists of items. | javascript var fruits = ["apple", "banana", "orange"]; |
Objects | Collections of key-value pairs, representing complex data structures. | javascript var person = { name: "John", age: 30, isStudent: false }; |
Functions | Reusable blocks of code that can perform tasks or return values. | javascript function add(a, b) { return a + b; } |
Date and Time (Date object) | Provides functionality for working with dates and times. | javascript var today = new Date(); console.log(today.getDate()); |
Sets | Collections of unique values, useful for managing unique elements. | javascript var uniqueNumbers = new Set([1, 2, 3, 3, 4, 4]); |
Maps | Collections of key-value pairs, similar to objects but with more features. | javascript var userMap = new Map(); userMap.set("name", "Alice"); |
Functions and Closures
Concept | Description | Example |
---|---|---|
Functions | Reusable blocks of code that can perform tasks or computations. | javascript function greet(name) { console.log("Hello, " + name + "!"); } |
Function Expressions | Functions defined as expressions and can be assigned to variables or passed as arguments. | javascript var greet = function(name) { console.log("Hello, " + name + "!"); }; |
Arrow Functions (ES6) | A concise way to define functions using the => syntax. |
javascript const greet = (name) => console.log(`Hello, ${name}!`); |
Closures | Functions that “remember” and have access to their outer (enclosing) function’s variables. | javascript function outerFunction() { var outerVar = "I am from outer function"; function innerFunction() { console.log(outerVar); } return innerFunction; } |
Advanced JavaScript Concepts
1. Object-Oriented Programming (OOP):
- JavaScript supports object-oriented programming principles, including the creation of classes and objects. You can define constructors, use prototypes, and implement inheritance.
2. Prototypes and Inheritance:
- JavaScript uses prototypal inheritance, where objects can inherit properties and methods from other objects. Understanding prototypes and how inheritance works is crucial for designing efficient and extensible code.
3. Closures and Scope:
- Advanced understanding of closures and scope helps in managing variable lifecycles and creating private variables. Closures are commonly used for data encapsulation.
4. Promises and Asynchronous Programming:
- Promises are used to handle asynchronous operations in a more structured way. Understanding how to work with promises, async/await, and callback functions is essential for managing non-blocking code.
Conclusion
In conclusion, JavaScript is a versatile and powerful programming language that plays a central role in web development and beyond. From its humble beginnings as a scripting language for web browsers, JavaScript has evolved into a feature-rich language with a wide range of applications. Understanding both the fundamentals and advanced concepts of JavaScript is essential for developers to harness its full potential.
From manipulating the Document Object Model (DOM) to creating closures, working with asynchronous operations, and employing design patterns, JavaScript offers a vast array of tools and techniques for building interactive and efficient applications. It’s not limited to web development; JavaScript is also used in server-side programming, mobile app development, and even in emerging technologies like IoT (Internet of Things).
FAQs
JavaScript is a high-level, interpreted scripting language used for web development. It adds interactivity and dynamic features to websites, allowing for client-side scripting.
No, JavaScript and Java are two different programming languages with different syntax, use cases, and purposes. They share a similar name due to marketing decisions but are not related.
JavaScript can be used in web browsers to enhance the functionality of web pages. It can also be used on the server-side with technologies like Node.js. Additionally, JavaScript is used for building mobile apps, desktop applications, and more.
The Document Object Model is a programming interface for web documents. It represents the page so that programs can change the document structure, content, and style dynamically.
Read Also
Related posts:
- AMC Full Form: Benefits, Components, Needs, Advantage
- ORS Full Form: Dehydration, Myths, Flavors, Varieties & Facts
- PCC Full Form: Importance, Types, Application Process
- PAN Full Form: Legal Provisions, Regulations,
- BRB Full Form: Productive, Routine, Distractions
- MCD Full From: Introduction, Responsibility, Challenges
- CT Scan Full Form: Scans, price, Advantages
- USA Full Form: History, Economics,Technology, culture