JavaScript Object Notation (JSON) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is often used to transmit data between a server and a web application, as an alternative to XML.
Introduction to JSON
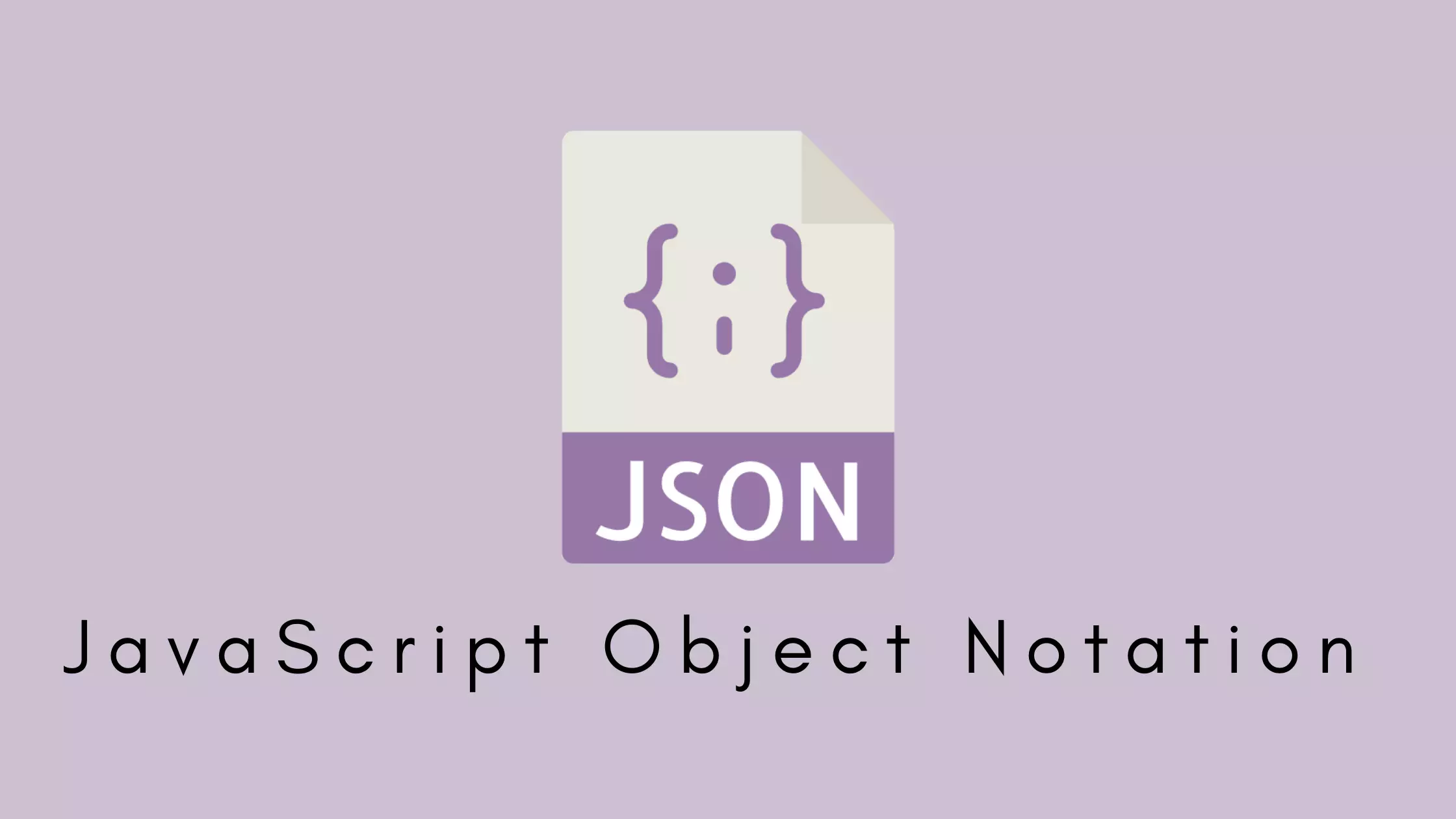
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format that has become a ubiquitous part of modern web development. It serves as a structured way to represent and exchange data between different systems. JSON is easy for humans to read and write and easy for machines to parse and generate, making it an ideal choice for data storage and transmission in various applications.
Key Characteristics of JSON:
- Data Structure: JSON represents data in a structured format using key-value pairs. It consists of objects, arrays, strings, numbers, booleans, and null values.
- Human-Readable: JSON is designed to be easily readable by humans, which makes it an excellent choice for configuration files and data storage that may need occasional manual editing.
- Language Agnostic: While it originated as a subset of JavaScript, JSON is not tied to any specific programming language. It’s supported by a wide range of programming languages, making it a universal data format for data interchange.
JSON Syntax
Element | Syntax | Description |
---|---|---|
Object | {} | Represents a collection of key-value pairs. |
Key-Value Pair | “Key” : value | Associates a key (string) with a value. |
Key | “Key” | A string enclosed in double quotes. |
Value | String, Number, Boolean, Object, Array, Null | Represents the data associated with a key. Can be a string, number, boolean, object, array, or null value. |
Array | [] | Represents an ordered list of values. |
String | “Value” | A sequence of characters enclosed in double quotes. |
Number | 42 or 3.1416 | Can be an integer or a floating-point number. |
Boolean | true or false | Represents true or false values. |
Null | null | Represents a null value. |
Creating JSON Data
Method | Description | Example |
---|---|---|
Literal Notation | Directly define JSON data using curly braces {}. | json { “name”: “John Doe”, “age”: 30, “city”: “New York” } |
JavaScript Object | Create a JavaScript object and convert it to JSON using `JSON.stringify()`. | javascript const person = { name: “John Doe”, age: 30, city: “New York” }; const jsonPerson = JSON.stringify(person); |
Array of Objects | Represent an array of objects within square brackets []. | json [ { “name”: “John Doe”, “age”: 30 }, { “name”: “Jane Smith”, “age”: 28 } ] |
Using `JSON.stringify()` | Build a JavaScript object and convert it to a JSON string. | javascript const data = { name: “John Doe”, age: 30, city: “New York” }; const jsonData = JSON.stringify(data); |
Accessing JSON Data
Method | Description | Example |
---|---|---|
Dot Notation | Access properties of JSON objects using dot notation. | javascript const person = { name: “John Doe”, age: 30, address: { city: “New York”, postalCode: “10001” } }; const name = person.name; // Accessing the name property const city = person.address.city; // Accessing a nested property |
Bracket Notation | Access properties using bracket notation, especially useful for property names with spaces or special characters. | javascript const person = { “full name”: “John Doe”, age: 30, “home address”: { city: “New York”, “postal code”: “10001” } }; const fullName = person[“full name”]; // Accessing a property with spaces const postalCode = person[“home address”][“postal code”]; // Accessing nested properties with spaces |
Array Indexing | Access elements in JSON arrays using index notation. | javascript const fruits = [“apple”, “banana”, “cherry”]; const secondFruit = fruits[1]; // Accessing the second element (index 1) |
Modifying JSON Data
Operation | Description | Example |
---|---|---|
Adding Properties | Add new key-value pairs to a JSON object. | javascript const person = { name: “John Doe”, age: 30 }; person.city = “New York”; // Adding a new property |
Updating Values | Change the values of existing properties. | javascript const person = { name: “John Doe”, age: 30 }; person.age = 31; // Updating the age property |
Removing Properties | Delete key-value pairs from a JSON object. | javascript const person = { name: “John Doe”, age: 30 }; delete person.age; // Removing the age property |
Array Operations | Manipulate elements within a JSON array. | javascript const fruits = [“apple”, “banana”, “cherry”]; fruits.push(“orange”); // Adding an element fruits[1] = “kiwi”; // Updating an element fruits.pop(); // Removing the last element |
Complex Modifications | Modify nested data structures in JSON objects. | javascript const person = { name: “John Doe”, address: { city: “New York”, postalCode: “10001” } }; person.address.city = “Los Angeles”; // Updating a nested property |
JSON Best Practices
- Consistent Data Structure: Maintain a consistent data structure within your JSON objects or arrays. This makes it easier to parse and work with the data consistently.
- Use Descriptive Key Names: Choose clear and descriptive key names that convey the purpose of the data. Avoid using cryptic or overly short key names.
- Nesting Wisely: Use nested objects and arrays when it logically represents the data structure. However, avoid excessive nesting, which can make the JSON structure hard to read and work with.
- Use Arrays for Lists: Use JSON arrays to represent lists of similar items (e.g., a list of products, comments, or user preferences).
- Avoid Circular References: Ensure that there are no circular references in your JSON data structures, as this can cause issues when serializing and deserializing.
- Use Double Quotes for Key Names and Strings: Always enclose key names and string values in double quotes (” “) as per the JSON specification.
Working with JSON in Web APIs
- JSON as the Data Format: When designing a web API, consider using JSON as the default data format for both request payloads and response data. It’s easy to work with in most programming languages.
- Sending JSON Data (Client to Server):
- When sending data to a server in a JSON format, use the HTTP method POST or PUT. You typically include the JSON data in the request body.
- Example using JavaScript and the Fetch API:
const data = { name: “John Doe”, age: 30 }; fetch(‘https://api.example.com/resource’, { method: ‘POST’, headers: { ‘Content-Type’: ‘application/json’ }, body: JSON.stringify(data) });
- Receiving JSON Data (Server to Client):
- When receiving JSON data from a server, ensure that the server sets the Content-Type header to application/json to indicate that the response contains JSON data.
- Example using JavaScript and Fetch API:
fetch(‘https://api.example.com/resource’) .then(response => response.json()) .then(data => { // Work with the JSON data console.log(data.name); });
- Error Handling: Handle errors gracefully when working with JSON data. Servers often provide error responses in JSON format, so be prepared to parse and handle them appropriately.
- Authentication and Authorization: Secure your web API endpoints using authentication and authorization mechanisms. JSON Web Tokens (JWT) are a common choice for secure authentication.
- Documentation: Provide comprehensive documentation for your API, including examples of the JSON data format for requests and responses. This helps developers understand how to interact with your API.
Conclusion
In conclusion, JSON (JavaScript Object Notation) is a versatile and widely adopted data interchange format that plays a crucial role in modern web development and data exchange. Its simplicity, readability, and compatibility across various programming languages make it a popular choice for transmitting and storing data.
FAQs
JSON stands for “JavaScript Object Notation.”
JSON is used for data interchange between a server and a client, data storage, configuration files, and representing structured data in a human-readable format.
JSON supports several data types, including strings, numbers, booleans, null, objects, and arrays.
{}
for objects or square brackets [] for arrays. You can also use JSON.stringify() to convert JavaScript objects into JSON strings.Read Also
Related posts:
- AMC Full Form: Benefits, Components, Needs, Advantage
- ORS Full Form: Dehydration, Myths, Flavors, Varieties & Facts
- PCC Full Form: Importance, Types, Application Process
- PAN Full Form: Legal Provisions, Regulations,
- BRB Full Form: Productive, Routine, Distractions
- MCD Full From: Introduction, Responsibility, Challenges
- CT Scan Full Form: Scans, price, Advantages
- USA Full Form: History, Economics,Technology, culture